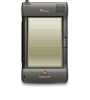
AppleToTheCore
Check out my vintage Apple collection.
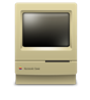
RescueMyClassicMac
Do you need old system software disks to boot your vintage Mac?
#include <OneWire.h> int DS18S20_Pin = 9; OneWire ds(DS18S20_Pin); byte digit0 = 10; byte digit1 = 11; int xyz = 0; float temperature; int nnn; int fTemp; byte sevenSegmentPins[] = {2,3,4,5,6,7,8}; byte sevenSegment[10][7] = { //a b c d e f g { 0,0,0,0,0,0,1 }, // 0 { 1,0,0,1,1,1,1 }, // 1 { 0,0,1,0,0,1,0 }, // 2 { 0,0,0,0,1,1,0 }, // 3 { 1,0,0,1,1,0,0 }, // 4 { 0,1,0,0,1,0,0 }, // 5 { 0,1,0,0,0,0,0 }, // 6 { 0,0,0,1,1,1,1 }, // 7 { 0,0,0,0,0,0,0 }, // 8 { 0,0,0,1,1,0,0 } // 9 }; void setup() { pinMode(digit0, OUTPUT); //pin 10 pinMode(digit1, OUTPUT); //pin 11 for(int i=0; i<7; i++) { pinMode(sevenSegmentPins[i], OUTPUT); } digitalWrite(digit0, HIGH); digitalWrite(digit1, HIGH); temperature = getTemp(); fTemp = (temperature * 1.8) + 32.0; // Convert Celcius to Fahrenheit nnn = fTemp*100; } void segmentWrite(byte digit) { byte pin = 2; for (byte i=0; i<7; ++i) { digitalWrite(pin, sevenSegment[digit][i]); ++pin; } } void loop() { xyz++; if (xyz == 1000) { xyz = 0; temperature = getTemp(); int fTemp = (temperature * 1.8) + 32.0; // Convert Celcius to Fahrenheit Serial.println(fTemp); } showNum(nnn); } void showNum(int n) { //display digit 0 int n1 = (n%10000)/1000; //digit 1 int n2 = (n%1000)/100; digitalWrite(digit0, LOW); //enable digit0 segmentWrite(n2); //send number to the segments delay(10); // delay digitalWrite(digit0, HIGH); //disable digit0 digitalWrite(digit1, LOW); //enable digit1 segmentWrite(n1); //send number to the segments delay(10); // delay digitalWrite(digit1, HIGH); //disable digit1 } float getTemp(){ //returns the temperature from one DS18S20 in DEG Celsius byte data[12]; byte addr[8]; if ( !ds.search(addr)) { //no more sensors on chain, reset search ds.reset_search(); return -1000; } if ( OneWire::crc8( addr, 7) != addr[7]) { Serial.println("CRC is not valid!"); return -1000; } if ( addr[0] != 0x10 && addr[0] != 0x28) { Serial.print("Device is not recognized"); return -1000; } ds.reset(); ds.select(addr); ds.write(0x44,1); // start conversion, with parasite power on at the end byte present = ds.reset(); ds.select(addr); ds.write(0xBE); // Read Scratchpad for (int i = 0; i < 9; i++) { // we need 9 bytes data[i] = ds.read(); } ds.reset_search(); byte MSB = data[1]; byte LSB = data[0]; float tempRead = ((MSB << 8) | LSB); //using two's compliment float TemperatureSum = tempRead / 16; return TemperatureSum; }